スクリーンショット
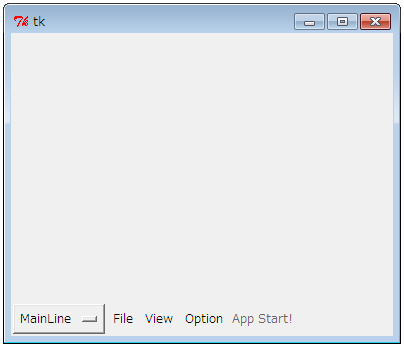
とりあえずこんな感じでいいんじゃないかなぁ、と。出来るだけシンプルに。
MainLine・・・線の種類です。補助線もあったらいいかなっと思って。
File・・・・・ファイル操作メニュです。開くとか保存とか。
View・・・・・画面操作メニュです。全体表示とか。
Option・・・・設定メニュです。デフォルトの線の太さとか。
後は基本、ショートカットキーもしくは右クリックでポップアップメニュで操作するスタイルに
する予定。
ソース
通常のメニューやオプションメニュになんとなく必要そうな項目だけを、ラベルだけ加えました。
メソッドとの関連付けは後ほど。
マウス操作とキーボードのバインディングも空っぽだけど、やり方を忘れないように書きます。
- # -*- coding: utf-8 -*-
- import tkinter as tk
-
- class DrawApp():
-
- def __init__(self):
- self.window = tk.Tk()
- self.set_gui()
- self.reset_bind()
-
- def mainloop(self):
- self.window.mainloop()
-
- def set_gui(self):
- # canvas
- self.canvas = tk.Canvas(self.window, bg="white")
- self.canvas.pack(fill=tk.BOTH,expand=True)
-
- # bottom menu
- self.menu = tk.Frame(self.window)
- self.menu.pack(fill=tk.BOTH)
-
- # line type menu
- LINES = ['MainLine','SubLine','Arrow']
- self.line_type = tk.StringVar()
- self.line_type.set(LINES[0])
- line_menu = tk.OptionMenu(self.menu, self.line_type, *LINES)
- line_menu.pack(side=tk.LEFT)
-
- # file menu
- file_btn = tk.Menubutton(self.menu,text='File')
- menu = tk.Menu(file_btn,tearoff=False)
- menu.add_command(label='Open...')
- menu.add_command(label='Save...')
- menu.add_command(label='Import')
- menu.add_command(label='Export')
- file_btn.configure(menu=menu)
- file_btn.pack(side=tk.LEFT)
-
- # view menu
- view_btn = tk.Menubutton(self.menu,text='View')
- menu = tk.Menu(view_btn,tearoff=False)
- menu.add_command(label='Zoom')
- menu.add_command(label='View All')
- menu.add_command(label='Backgraund Image...')
- view_btn.configure(menu=menu)
- view_btn.pack(side=tk.LEFT)
-
- # option menu
- option_btn = tk.Menubutton(self.menu,text='Option')
- menu = tk.Menu(option_btn,tearoff=False)
- menu.add_command(label='Setting')
- option_btn.configure(menu=menu)
- option_btn.pack(side=tk.LEFT)
-
- # message label
- self.msg = tk.StringVar()
- msg_label = tk.Label(self.menu,textvariable=self.msg,fg='#666666')
- msg_label.pack(side=tk.LEFT)
-
- self.show_message('App Start!')
-
- def show_message(self,message):
- self.msg.set(message)
-
- def set_bind(self):
- self.remove_bind()
-
- self.canvas.bind('<ButtonPress-1>', self.on_left_press)
- self.canvas.bind('<ButtonPress-2>', self.on_center_press)
- self.canvas.bind('<ButtonPress-3>', self.on_right_press)
- self.canvas.bind('<Shift-ButtonPress-3>', self.on_right_press)
- self.canvas.bind('<B1-Motion>', self.on_left_motion)
- self.canvas.bind('<B2-Motion>', self.on_center_motion)
- self.canvas.bind('<B3-Motion>', self.on_right_motion)
- self.canvas.bind('<ButtonRelease-1>', self.on_left_release)
- self.canvas.bind('<ButtonRelease-2>', self.on_center_release)
- self.canvas.bind('<ButtonRelease-3>', self.on_right_release)
- self.canvas.bind('<Motion>',self.on_motion)
- self.window.bind('<KeyPress>',self.on_key_press)
- self.window.bind('<Control-KeyPress>',self.on_key_pressCtrl)
- self.window.bind('<Alt-KeyPress>',self.on_key_pressAlt)
- self.window.bind('<MouseWheel>',self.on_mouse_wheel)
-
- def remove_bind(self):
- self.canvas.unbind("<ButtonPress-1>")
- self.canvas.unbind('<ButtonPress-2>')
- self.canvas.unbind('<ButtonPress-3>')
- self.canvas.unbind('<B1-Motion>')
- self.canvas.unbind('<B2-Motion>')
- self.canvas.unbind('<B3-Motion>')
- self.canvas.unbind('<ButtonRelease-1>')
- self.canvas.unbind('<ButtonRelease-2>')
- self.canvas.unbind('<ButtonRelease-3>')
- self.window.unbind('<KeyPress>')
- self.window.unbind('<KeyRelease>')
-
- def on_motion(self,event):
- return
-
- def on_left_press(self,event):
- return
-
- def on_left_motion(self,event):
- return
-
- def on_left_release(self,event):
- return
-
- def on_right_press(self,event):
- return
-
- def on_right_motion(self,event):
- return
-
- def on_right_release(self,event):
- return
-
- def on_center_press(self,event):
- return
-
- def on_center_motion(self,event):
- return
-
- def on_center_release(self,event):
- return
-
- def on_key_press(self,event):
- return
-
- def on_key_pressCtrl(self,event):
- return
-
- def on_key_pressAlt(self,event):
- return
-
- def on_key_release(self,event):
- return
-
- def on_mouse_wheel(self,event):
- return
-
-
- def main():
- app = DrawApp()
- app.mainloop()
-
-
- if __name__ == '__main__':
- main()